DOM is an acronym for Document Object Model, commonly used in web development. DOM manipulation is the process of using JavaScript to add, remove, and modify elements of a website. It is a very common practice and a crucial part of web development.
The DOM (Document Object Model) can be manipulated to create web applications that update data and layout without refreshing the page. Items can be deleted, moved, or rearranged throughout the document.
Definition of the DOM and Basic concepts
DOM, which stands for Document Object Model, is used in JavaScript to manipulate the webpage. The DOM is a tree-like structure that shows the hierarchical relationship between various HTML elements. It can be simply described as a tree of nodes generated by the browser. Each node has unique properties and methods that can be changed using JavaScript.
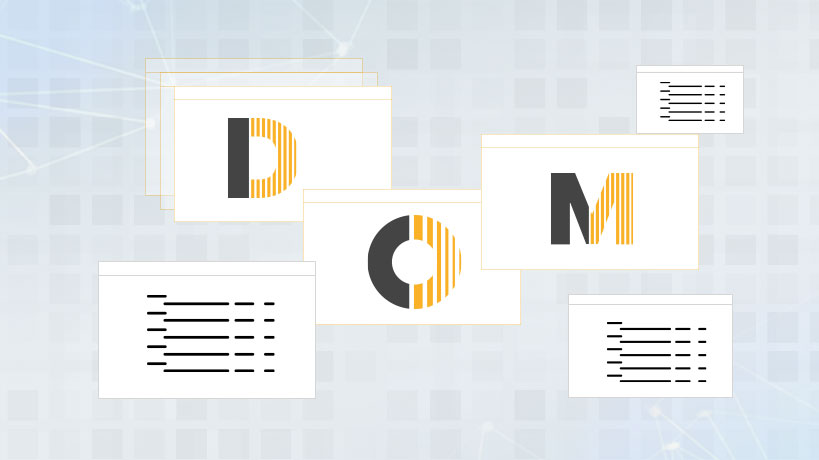
Examples of JavaScript DOM Manipulation
Following are some examples and sample codes of DOM manipulation:
Adding a new element to a web page:
document.getElementById(“myElement”).appendChild(document.createElement(“div”));
Removing an element from a web page:
document.getElementById(“myElement”).parentNode.removeChild(document.getElementById(“myElement”));
Changing the text of an element:
document.getElementById(“myElement”).innerHTML = “Hello, world!”;
Reordering elements on a web page:
var myElements = document.getElementsByTagName(“div”);
myElements[0].parentNode.insertBefore(myElements[1], myElements[0]);
DOM manipulation is a powerful tool that can be used to create interactive and dynamic web pages.
DOM Tree Analogy
Think about the DOM as a tree. Root of this tree is the document itself, i.e. the web page. Each node of this tree represents a different element or sections in the document. The branches that connect the nodes to each other are relationships like parent-child relationships. So, each child node is residing within its parent node. Each child node has a parent node except for the root node, which has no parent node.
The DOM tree analogy can be helpful for understanding DOM JavaScript manipulation. For example, if you want to change the text of a paragraph, you would find the paragraph node in the DOM tree and then change its textContent property.
DOM tree importance in DOM manipulation JavaScript?
The importance of understanding the DOM tree is essential. For example, if you want to change the text of a button, you would first find the button element in the DOM tree. Then, you would use the textContent property to set the new text for the button.
DOM tree & DOM methods are important for understanding how change in one element affects other elements on the page. For example, if you add a new element to the DOM tree, all of the elements below it in the tree will be moved down one position.
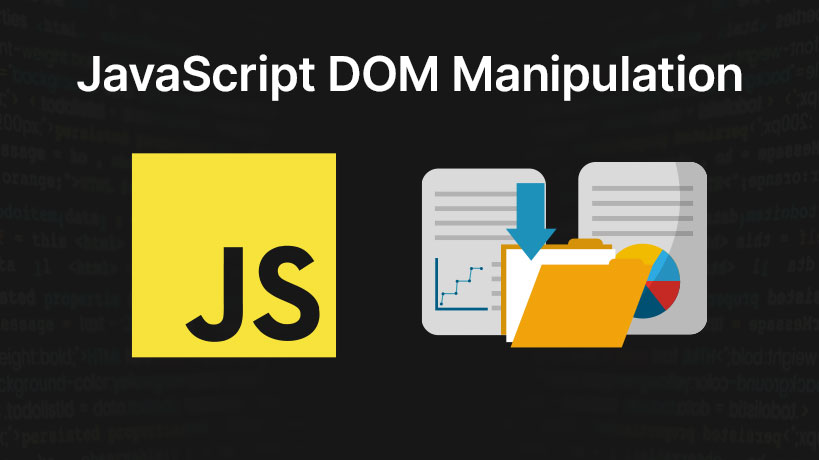
Overall, the DOM tree is a valuable tool for manipulating the elements on a web page. It provides a way to access and modify elements in a structured and organized way.
Traversing the DOM
Traversing the DOM is the process of accessing and manipulating the nodes in a Document Object Model (DOM). The update, replace, or edit can be done via JavaScript dom manipulation
There are several ways to traverse the DOM. One way is to use the DOM methods getElementById() and getElementsByTagName(). These methods return a reference to a specific element or all elements of a particular type.
Another way to traverse the DOM is to use the DOM methods parentNode, firstChild, nextSibling, and previousSibling. These methods return the parent node, first child node, next sibling node, and previous sibling node of the current node.
Traversing the DOM is a fundamental skill for working with HTML and XML documents. It is used in many different tasks, such as adding and removing elements, changing the properties of elements, and event handling.
To traverse the DOM from parent to child and sister nodes, you can use the following steps:
- Start at the root node of the DOM.
- Get the children of the current node.
- For each child node, do the following:
- Get the children of the child node.
- Repeat step 3 until there are no more child nodes.
- Repeat step 2 until there are no more child nodes of the root node.
Here is an example of how you would traverse the DOM from parent to child and sister nodes in JavaScript:
var root = document.getElementById(“root”);
var children = root.children;
for (var i = 0; i < children.length; i++) {
var child = children[i];
var childChildren = child.children;
for (var j = 0; j < childChildren.length; j++) {
var childChild = childChildren[j];
console.log(childChild);
}
}
Similarly, TS DOM and React DOM manipulation are ways to traverse and access the DOM.
TS DOM, Test Suites DOM, consist of tests for each level of the DOM specification, represented in XML. React DOM manipulation is simply manipulating the elements through DOM in React.js.
Conclusion
DOM manipulation in javascript is the process of interacting with the DOM API to change or modify an HTML document that will be displayed in a web browser. By manipulating the DOM, we can create web applications that update the data in a web page without refreshing the page.
The DOM stands for Document Object Model. The Document Object Model (DOM) is a tree-like structure illustrating the hierarchical relationship between various HTML elements.
In order to change or modify an element in the DOM, you need to select that specific element. Thus, JavaScript has six methods to select an element from a document. We can navigate through the tree nodes and access them using node relationships like a parent, children, siblings, etc. We have learned how to get the parents, children, and siblings.
In dom manipulation in javascript, A node can have more than one child. Siblings are nodes with the same parent like brothers or sisters. Except for the top node, which has no parent, each node has exactly one parent.
We have discussed How to Traverse and Move Around the DOM, and How to Manipulate Elements in the DOM. We have also seen working with Events and Manipulating Element’s Styles and different Scripting Web Forms with examples.